Octo in CJS
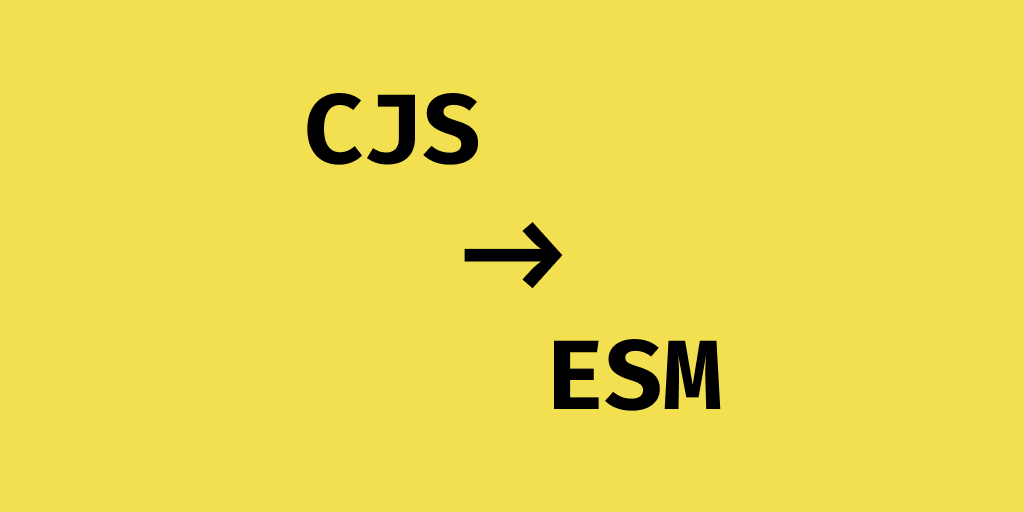
In Node, there are now two kinds of scripts: there are old-style CommonJS (CJS) scripts and new-style ESM scripts (aka MJS). CJS scripts use require() and module.exports; ESM scripts use import and export.
Octo exclusively supports ESM modules, requiring "type": "module"
in package.json.
In this article we will explore how to modify Octo imports to make them work in CJS.
- ESM
- CJS
import { App, LocalStateProvider } from '@quadnix/octo';
import { OctoAws, RegionId, S3StaticWebsiteService } from '@quadnix/octo-aws-cdk';
import { dirname, join } from 'path';
import { fileURLToPath } from 'url';
const __dirname = dirname(fileURLToPath(import.meta.url));
(async () => {
const { App, LocalStateProvider } = await import('@quadnix/octo');
const { OctoAws, RegionId, S3StaticWebsiteService } = await import('@quadnix/octo-aws-cdk');
const { join } = await import('path');
// __dirname is predefined in CJS.
})();
Take a look at how this code is written in ESM and compare it to the adjustments needed for CJS. Notice the use of IIFE in CJS to support Octo imports asynchronously.